Object-Oriented Programming (OOP) is a popular way to write computer programs. This concept is used by many programmers because it is making code easier to understand and manage. But what exactly is OOP, and why is it so important? Let’s explore this topic in a simple and detailed way that even a ninth grader can understand.
Understanding the Basics
Objects and Classes
In OOP, the two most important concepts are objects and classes.
- Objects: Think of an object as a real-world thing. It can be anything like a car, a dog, or a book. Each object has its own properties (like colour, size, and name) and behaviours (like drive, bark, and open).
- Classes: A class is like a blueprint for creating objects. For example, a car class would be a blueprint for making different cars. The class defines what properties and behaviours the objects will have.
Example:
Imagine you have a class called Car. This class has properties like colour, brand, and model. It also has behaviours like start, stop, and drive. When you create an object from this class, like a red Toyota Corolla, you are making an instance of the Car class.
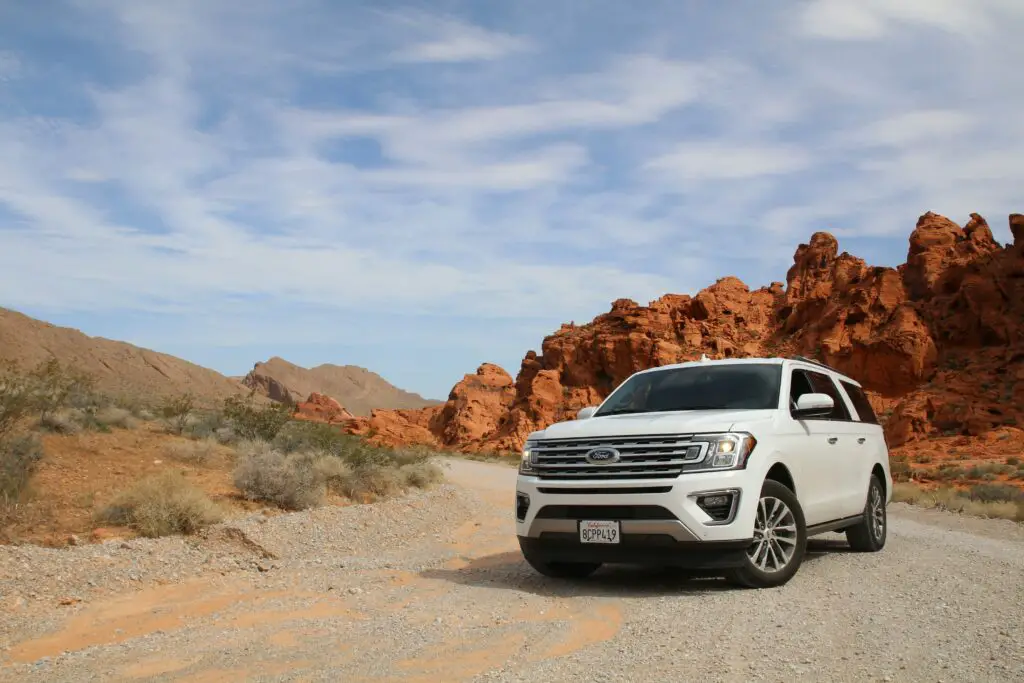
Key Principles of OOP
OOP has four main principles: Encapsulation, Inheritance, Polymorphism, and Abstraction. Let’s break these down.
1. Encapsulation
Encapsulation is about keeping the details inside an object hidden from the outside. Think of it like a capsule that holds the medicine. You only see the outer shell, not the contents inside.
- Example: When you use a TV remote, you press the buttons to change channels or adjust the volume, but you don’t need to know how the remote sends signals to the TV. The internal workings are hidden from you.

2. Inheritance
Inheritance allows one class to inherit properties and behaviours from another class. This is helps to reuse code and make it easier to create new objects.
- Example: If you have a class called Animal with properties like name and age, and behaviours like eat and sleep, you can create a new class called Dog that inherits from Animal. The Dog class will automatically have the name, age, eat, and sleep features, and you can add more specific features like bark.

3. Polymorphism
Polymorphism means “many forms.” In OOP, it allows objects to be treated as instances of their parent class rather than their actual class. This means a single function can work in different ways depending on the object it is acting upon.
- Example: If you have a function called make Sound that is used by both the Dog and Cat classes, the Dog object might bark while the Cat object might meow. The same function works differently based on the object.

4. Abstraction
Abstraction is about hiding the complex details and showing only the essential features of an object.
- Example: When you drive a car, you use the steering wheel, pedals, and gear stick without needing to know how the engine works. The complex details are hidden, and you interact only with the necessary parts.
Benefits of Object-Oriented Programming
OOP offers many advantages:
- Easy to Understand: By breaking down a program into objects, it becomes easier to understand and manage.
- Reusability: Code can be reused across different parts of the program or even in different programs.
- Flexibility: Changes in one part of the program do not heavily affect other parts.
- Maintenance: Easier to find and fix bugs since the code is organized around objects.
In conclusion Object-Oriented Programming is basic concept write computer programs by using the objects and classes. It uses these 4 main principles that are, encapsulation, inheritance, polymorphism, and abstraction. This 4 OOP concept is useful to make code easier to manage, understand, and reuse. Even if you are just starting out with programming, understanding these basics of OOP will help you write better and more efficient programs. Happy coding!