The JavaScript map
function is a common syntax used in programming to transform arrays. If you are working with JavaScript and want to handle arrays efficiently, understanding the JavaScript map
function is crucial. This article will explain what is the map
function, how it works, and provide examples to help you understand it more easily.
What is the JavaScript map
Function?
The JavaScript map
function creates a new array populated with the results of calling a provided function on every element in the calling array. In simpler terms, it takes an array, does something to each item, and returns a new array with the updated items.
How Does the JavaScript map
Function Work?
The map
function takes a callback function as an argument. This callback function executed on each element of a array, and result is a collected into a new array. Here is the syntax,
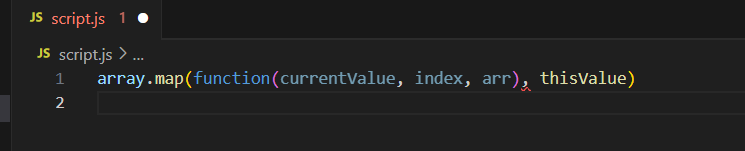
currentValue
is the current element being processed in the array.index
(optional) is the index of the current element.arr
(optional) is the arraymap
was called upon.thisValue
(optional) is the value to use asthis
when executing the callback.
Example of the JavaScript map
Function
Let’s look at a basic example to illustrate how the JavaScript map
function works.
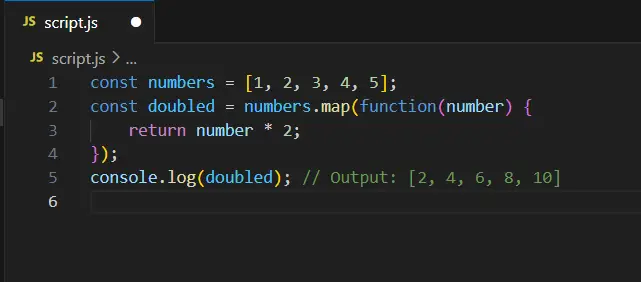
In this example, the map
function multiplies each number in the numbers
array by 2, creating a new array doubled
.
Benefits of Using the JavaScript map
Function
- Immutability: The
map
function does not change the original array. It returns a new array, which helps avoid the other side effects. - Conciseness: Using the
map
function can make your code shorter and more readable compared to using traditional loops. - Chaining: Since
map
returns a new array, you can chain it with other array methods likefilter
andreduce
.
Practical Uses of the JavaScript map
Function
The JavaScript map
function can be used in various scenarios:
- Transforming Data: If you need to transform data in an array, such as converting all strings to uppercase or formatting dates, the
map
function is ideal. - Extracting Data: You can use
map
to extract specific properties from an array of objects.
These are the example of extracting data,
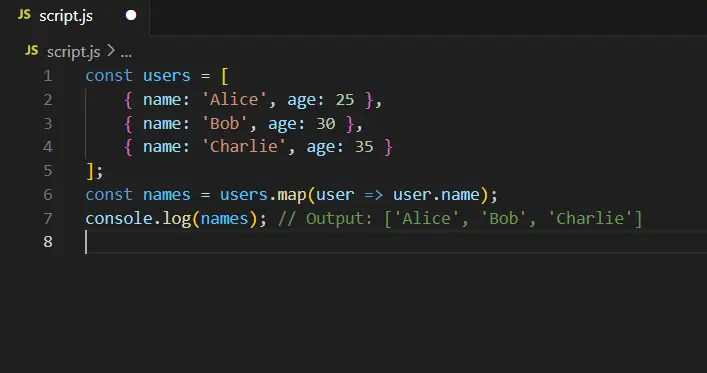
In this example, we use the map
function to create new array containing only the names of the users.
In conclusion JavaScript map
function is an essential tool for any JavaScript developer. It allow you to transform arrays eficiently and write the cleaner, more readable code. By understanding how JavaScript map
function works and applying it is in your projects, you can make your JavaScript code more effective.
Remember to practice using the JavaScript map
function in different scenarios to get comfortable with its syntax and capabilities. Happy coding!