Hello and welcome again to my blog sachiyatech. In this article we will look about interesting topic, In web development, understanding JavaScript concept is very important, especially when it comes to the concept of asynchronous programming. But what exactly mean of asynchronous JavaScript, and why does it matter so much in the web development? Let’s discuss about.
A Quick Look at what is Asynchronous JavaScript
Imagine surfing a web page that stuck whenever it needs to fetch data or do something complicated. Frustrating, right? Well, that’s where asynchronous JavaScript come to the picture. Unlike the traditional synchronous way of doing things, which does tasks one after another, asynchronous JavaScript lets developers run multiple tasks simultaneously. This means you can run parallel task at one time. It will increase the web page loading speed and responsiveness.
What’s the Difference of Synchronous vs. Asynchronous Programming?
Let’s look about what is the difference between this asynchronous and synchronous JavaScript. In synchronous programming, each task has to wait for the one before it to finish, which can slow down the entire process of the application. With asynchronous programming, tasks do not wait around they others finished whenever they’re ready, even if it is other tasks are still processing. That keeps things moving smoothly and prevent any one task from slowing down the whole process of a application.

Why asynchronous JavaScript use in web development.
In web development, where users expect seamless experiences from the website. Asynchronous JavaScript take a lot of work for done this task. It is helps websites and web apps feel responsive and snappy, even when their doing complex stuff behind a scenes. By doing tasks simultaneously, asynchronous JavaScript makes it is possible to create dynamic and interactive web experiences thats keep users engaged and improve overall quality of a web site.
Using asynchronous JavaScript developers can build more realistic website and apps. And also it will increase the application reliability using real time updates, smooth animations and fast responsiveness. Using this function it will increase user attraction for the application. So if you are exper in the web development or beginner very important to learn this concept for your future career. Now we will discuss about what is asynchronous JavaScript.

Actually what is asynchronous JavaScript?
To learn the concept of asynchronous JavaScript, let’s break it down into simple terms. Simply, asynchronous programming means doing tasks independently without waiting for others to finish. This is allows for smoother and faster execution of tasks, making applications more responsive and efficient in web development.
In JavaScript, handling asynchronous tasks is made possible through something called the event loop. This loop keeps an eye on pending tasks and manages them in a way that doesn’t disrupt the flow of the main program. It’s like traffic control system, ensure that everything move smoothly.
Callback function
Let we discuss about very important feature in asynchronous JavaScript it is the callback function. It’s a function that gets passed into another function and gets called once a specific task is done or an event occurs. Think of it as a reminder set for a some particular job. when that job is finished, the callback function move to handle the next steps.
This basics of asynchronous JavaScript is very useful for your future web development. By mastering this concepts, developers can create websites and applications that is more responsive for the users and application. So, whether you’re just beginner or expert in the web development, understand this with asynchronous JavaScript is a must be useful for building your application with high performance.
What is event loop ?
The event loop also crucial task in asynchronous JavaScript. In JavaScript, the event loop is like the conductor of an orchestra, orchestrating the flow of tasks and ensuring everything runs smoothly. Like that, it mechanism that is allows JavaScript to handle multiple tasks concurrently without blocking a execution of the other code.
Let me give sime simple example for how the event loop works, imagine a busy chef in a kitchen. The chef has several tasks to complete, such as the chop vegetables, burn sauces, and grilling meats. Instead of waiting for one task to finish before starting the next, the chef wants multiple tasks simultaneously. This parallel processing ensures that cooking process remains is efficient.

Like that in JavaScript, the event loop continuously checks for pending tasks in the callback queue and executes them one by one. It operates in a loop, constantly monitoring the call stack for synchronous tasks and handling asynchronous tasks as they complete. This seamless coordination ensures that even the complex operations, such as a fetching data from the server or animating elements on webpage, can occur without causing the delays or interruptions in user experience.
By understanding inner workings of the event loop, you can get idea into how JavaScript manages asynchronous tasks and maintains responsiveness in web applications. With this knowledge, they can optimize their code to the leverage event loop effectively, creating fast, responsive, and interactive experiences that attracts users and enhance overall quality of their digital products.
Promises in asynchronous JavaScript
Dealing with asynchronous tasks can sometimes feel like navigating through a maze, especially when faced with what developers call “callback hell” it like a messy tangle of nested callbacks. But don’t worry promises will prevent it, offering a neat and organized way to handle these challenges.
Promises are very helpful for developers struggling with asynchronous programming. They simplify process by provide the clear structure and easy to understand the syntax. At their core, promises represent the eventual outcome of an asynchronous task, whether it succeeds or fails. This helps to developers manage tasks without getting lost in layers of callbacks.
Creating a promise in JavaScript is easily. Developers use the Promise constructor, passing in a function that defines the asynchronous task. This function contains a code for tasks like fetching data or the processing user input. Once the task is done, the promise either resolves with a result or rejects with an error, making it easier to handle different scenarios.
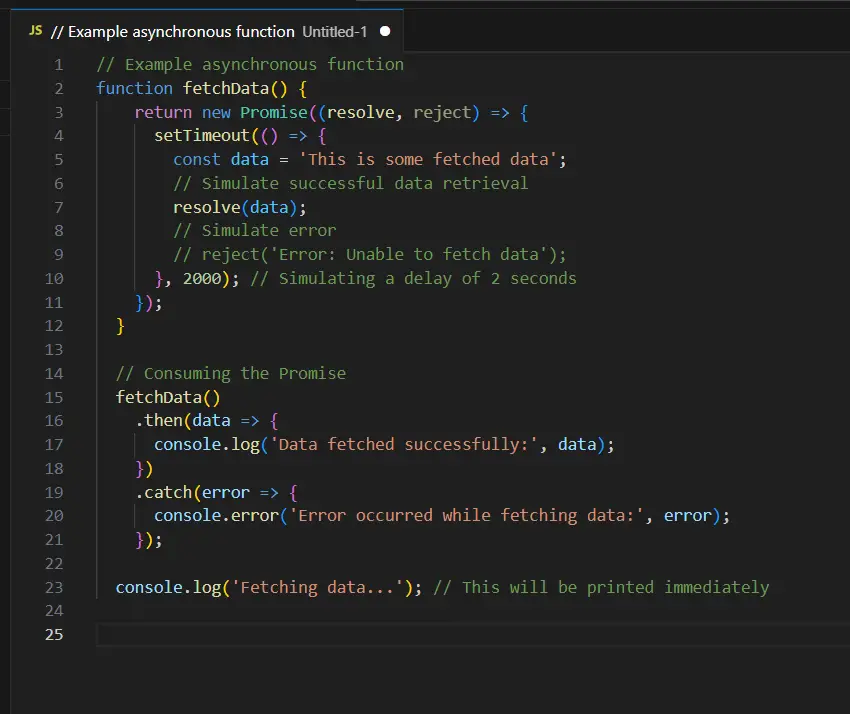
One great thing about promises is their ability to chain multiple tasks together. By using the then() method, developers can connect tasks in a sequence, ensuring each one waits for the previous one to finish. This streamlines code and makes it easier to follow, even when it dealing with the complex workflows.
If a promise is rejected, developers can use the catch() method to gracefully manage the error without causing chaos in the code. This robust error handling ensures applications remain stable and reliable, even in a challenging situations also.
Promises mark significant step forward in the JavaScript development, providing more organized and the manageable way to handle asynchronous tasks. With their clear syntax, support for chaining, and built-in error handling, promises ensure developers to write cleaner and more efficient code, in the web development.
Async/Await function
Async/await also another main function in JavaScript, making asynchronous programming simpler and more readable. It builds upon promises and offers thr best way to write code that deals with a asynchronous tasks.
At its core, async/await use around two keywords. It is async and await. When you declare a function as async, it means that function will handle asynchronous tasks. The await keyword is used inside async functions to pause the code execution until a promise is resolved, giving a more straightforward and sequential structure to the code.
Another advantage of async/await is its simple. Comparing promises, async/await makes code look more like regular synchronous code, which is easier to understand for many developers. It’s relief for those who find the promises and callbacks confusing.

Using async/await also use to make q clean code. It avoids the nesting or chaining of promises, resulting in a more straightforward and simple structure. This not only make a code easier to read but it reduces the chances of errors and makes the debugging simpler.
Error handling with async/await is also more straightforward. Instead of using separate blocks for success and errors, async/await allows developers to use try…catch blocks, which unify error handling and make the code more consistent and robust.
Overall, async/await is huge step forward in asynchronous programming. It combines a ease of writing synchronous code with handling asynchronous operations, opening up new possibilities for the building efficient and a maintainable JavaScript applications.
Let’s look about some examples for asynchronous JavaScript program.
Making asynchronous HTTP requests with the fetch API
The fetch API is use for making HTTP requests in JavaScript. Its asynchronous nature structure makes it ideal for fetching data from servers without blocking the execution of the other code. Here is simple example of it.

In this example, we define an async function fetchData() function to fetch data from an API endpoint. We use the await keyword to pause the function execution until the fetch operation is complete. This allows us handle a response asynchronously without block the main program.Next we will look about some another example.
Simulating asynchronous operations with setTimeout()
The setTimeout() function is example of the simulating asynchronous behavior in JavaScript. It allows us to execute the function after a specified delay, making it useful for scenarios like animations, polling, or handling asynchronous tasks. Here is how it works,
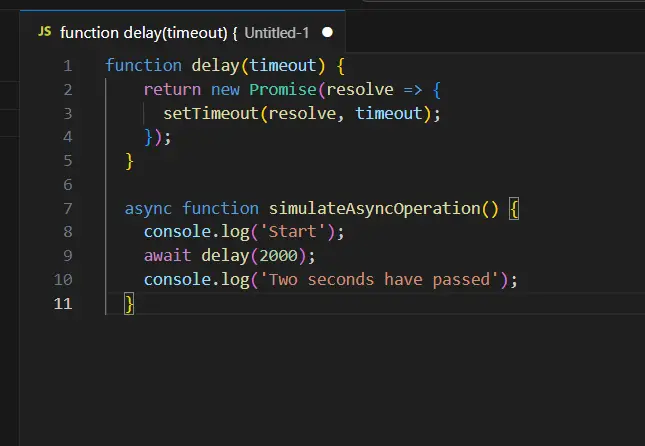
In this example, we define the delay() function that returns promise, which resolves after a specific timeout. We then use this function inside an async function simulateAsyncOperation() to simulate an asynchronous operation that takes two seconds to complete. The await keyword ensures that the code waits for delay before proceeding, demonstrating the asynchronous nature of a setTimeout().
These two examples are showcase how asynchronous JavaScript can be applied in a real-world scenarios, from fetching data from servers to simulating delays in the code execution. By understand and master this asynchronous concepts, developers can create a more efficient, responsive, and the user friendly web applications.
Best practices for improving asynchronous development
Firstly, avoid using blocking code whenever possible. Blocking code can make your application unresponsive because it stops other tasks from running while it waits for one task to finish. Instead, use asynchronous techniques like promises, async/await, or the event-driven methods. These methods allow to your application stay responsive and handle multiple tasks at once without a causing delays.
Next, managing concurrency is a important. Asynchronous operations can run concurrently, which is the great for performance. However, it not managed properly, it can be a lead to problems like the race conditions or the deadlocks. To avoid these issues, plan your asynchronous workflows carefully. Use synchronization tools like locks or semaphores when needed, and consider techniques like throttling or debouncing to control how quickly tasks are executed concurrently.
Lastly, handle errors in a correct manner. Errors can happen at the any stage of an asynchronous operation, from fetching data to process user input. Make sure to catch and handle errors effectively using tools like try…catch blocks or the error callbacks. Also, provide informative error messages and log errors to make debugging easier.

If you read this article, you’ve learned what about asynchronous JavaScript and why it is a important in web development. We covered topics like how asynchronous programming works, using promises and async/await to handle a asynchronous tasks, and practical examples of the tasks like fetching data and managing delays. We are also discussed some tips for writing better asynchronous code, like avoide anything that slows down the program, managing tasks running at the same time, and handling errors properly.
Using this knowledge, you’ll be better prepare to the write code that runs smoothly and efficiently, making your web applications is a more responsive and user friendly. So now the end of this article. If you have any problem regarding this please put it in the below comment section. And also read my other articles if you like. So good bye !! Happy coding !!